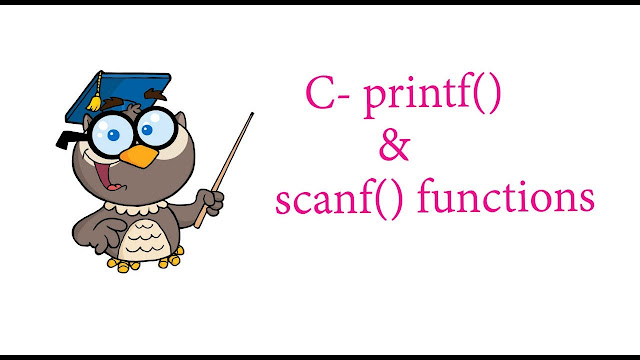
C printf( ) and scanf( ) Functions
- Any programming language needs to input/output the data from/to the user.
- In C printf( ) function is used to give the output on the console screen and scanf( ) function is used to take the input by the user.
- Printf( ) and scanf( ) function are inbuilt library functions which are included in "stdio.h" header file.
C programming is case-sensitive language.
That means, printf( ) and Printf( ) both are different. All characters in printf( ) and scanf( ) functions must be in lower case.
That means, printf( ) and Printf( ) both are different. All characters in printf( ) and scanf( ) functions must be in lower case.
printf() function:
In C programming language printf() function is used for giving the output to the console screen.
printf() function returns the characters taken by it. This function supports two type of characters.
- Ordinary Characters: These characters need to be placed within double qoutes " " and does not need any format specifier and can be printed directly without any conversions.
- Characters with Format Specifiers: These characters need format specifiers to tell the compiler in which format data needs to be displayed and conversion of data should be done by the compiler to the specified format.
Example:
➣ordinary characters :
➣Characters with format specifiers :
In this example we assgin the value "97 to a" but in printf we are using the format specifier "%c" which converts the value of integer into character. This is the reason why the output of printf is "a" instead of "97" because, the character equivalent of "97 is a".
➣another example of printf() with different format specifiers:
The various format specifiers are:
Format Specifiers | Description | Supported data types |
---|---|---|
%d | Signed integerint | short,unsigned short,int,long |
%hi | Signed integer(short) | short |
%hu | Unsigned integer(short) | unsigned short |
%l or %ld or %li | Signed integer | long |
%lu | Unsigned integer | unsigned int, unsigned long |
%lli,%lld | Signed integer(short) | long long |
%llu | Unsigned integer(short) | unsigned long long |
%o | Octal representation of integer(short) | short,unsigned short,int,unsigned int,long |
%x oor %X | Hexadecimal representation of Unsigned integer(short | short,unsigned short,int,unsigned int,long |
%c | Character | char,unsigned char |
%s | String | char* |
%e or %E | Scientific notatiom of float values | float,double |
%p | Address of pointer to void void* | void* |
%f | Floating point | float |
%lf | Floating point | double |
%Lf | Floating point | long double |
%g or %G | Similar as %e or %E | float,double |
%n | Prints nothing | |
%% | Prints % character |
scanf() function:
Scanf() function requires access over the address of the value entered.
"&" Ampersand is used for locating the address of the given input. It takes input from stdin.
To read any character, scanf() takes the help of format specifiers.
The format specifier %d is used in scanf() statement. So that, the value entered is received as an integer.
Ampersand is used before variable name “a” in scanf() statement as &a.
Example:
Ampersand is used before variable name “a” in scanf() statement as &a.
Example:
Some more programming examples with printf() and scanf():
Example2: program to print cube of a given number.
Interview questions on printf() and scanf() functions:
Q1. Mcq Type Questions:
(1)
#include<stdio.h>
#include<conio.h>
void main()
{
int a=5,b=6,c=11;
clrscr();
printf("%d %d %d");
getch();
}
What will output when you compile and run the above code?
(a)Garbage value garbage value garbage value
(b)5 6 11
(c)11 6 5
(d)Compiler error
Answer: (d)
(2)
#include<stdio.h>
void main()
{
clrscr();
printf("%d",printf("CQUESTIONBANK"));
getch();
}
What will output when you compile and run the above code?
(a)13CQUESTIONBANK
(b)CQUESTIONBANK13
(c)Garbage CQUESTIONBANK
(d)Compiler error
Answer: (b)
Explanation: Inner printf() will print first and then outer printf will display the length of the inner printf's string.
#include<stdio.h>
void main()
{
clrscr();
printf("%d",printf("CQUESTIONBANK"));
getch();
}
What will output when you compile and run the above code?
(a)13CQUESTIONBANK
(b)CQUESTIONBANK13
(c)Garbage CQUESTIONBANK
(d)Compiler error
Answer: (b)
(3)
#include<stdio.h>
#include<conio.h>
void main()
{
short int a=5;
clrscr();
printf("%d"+1,a);
getch();
}
What will output when you compile and run the above code?
(a)6
(b)51
(c)d
(d)Compiler error
Answer: (c)
#include<stdio.h>
#include<conio.h>
void main()
{
short int a=5;
clrscr();
printf("%d"+1,a);
getch();
}
What will output when you compile and run the above code?
(a)6
(b)51
(c)d
(d)Compiler error
Answer: (c)
(4)
#include<stdio.h>
int main()
{
printf("%d",5.00);
return 0;
}
What will be the output of the C program?
(a)run time error
(b)compile time error
(c)5
(d)0
Answer: (d)
Explanation: when we print the integer with .000 and many zero's as an extension then output will be 0.
(a)run time error
Q2. What is the return type of printf() and scanf() functions?
Ans. In C, printf() returns the number of characters successfully written on the output and scanf() returns number of items successfully read.
No comments: